Min element from Numpy Array: nan If you want to ignore the NaNs while finding the min values from numpy then use numpy.nanmin instead. Complete example is as follows. Get code examples like 'what is time complexity of minelement' instantly right from your google search results with the Grepper Chrome Extension.
In this article we will discuss how to find the minimum or smallest value in a Numpy array and it’s indices using numpy.amin().

Min_element C++ Stl
numpy.amin()
Python’s numpy module provides a function to get the minimum value from a Numpy array i.e.
Arguments :
- a : numpy array from which it needs to find the minimum value.
- axis : It’s optional and if not provided then it will flattened the passed numpy array and returns the min value in it.
- If it’s provided then it will return for array of min values along the axis i.e.
- If axis=0 then it returns an array containing min value for each columns.
- If axis=1 then it returns an array containing min value for each row.
Let’s look in detail,
Find minimum value & it’s index in a 1D Numpy Array:
Let’s create a 1D numpy array from a list i.e.
Find minimum value:
Now let’s use numpy.amin() to find the minimum value from this numpy array by passing just array as argument i.e.
Output:
It returns the minimum value from the passed numpy array i.e. 11
Find index of minimum value :
Get the array of indices of minimum value in numpy array using numpy.where() i.e.
Output:
In numpy.where() when we pass the condition expression only then it returns a tuple of arrays (one for each axis) containing the indices of element that satisfies the given condition. As our numpy array has one axis only therefore returned tuple contained one array of indices.
Find minimum value & it’s index in a 2D Numpy Array
Let’s create a 2D numpy array i.e.
Contents of the 2D numpy array are,
Find min value in complete 2D numpy array
To find minimum value from complete 2D numpy array we will not pass axis in numpy.amin() i.e.
It will return the minimum value from complete 2D numpy arrays i.e. in all rows and columns.
Find min values along the axis in 2D numpy array | min in rows or columns:
If we pass axis=0 in numpy.amin() then it returns an array containing min value for each column i.e.
Output:
If we pass axis = 1 in numpy.amin() then it returns an array containing min value for each row i.e.
Output:
Find index of minimum value from 2D numpy array:
Contents of the 2D numpy array arr2D are,
Let’s get the array of indices of minimum value in 2D numpy array i.e.
Output:
numpy.amin() & NaN
numpy.amin() propagates the NaN values i.e. if there is a NaN in the given numpy array then numpy.amin() will return NaN as minimum value. For example,
Output:
If you want to ignore the NaNs while finding the min values from numpy then use numpy.nanmin() instead.
Min_element Is Not A Member Of Std
Complete example is as follows,
Output
Min_element C++
Related Posts:
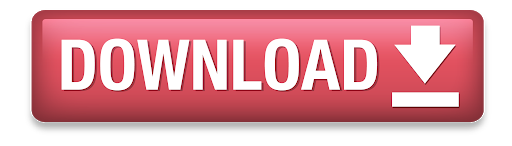